JavaScript, a web development language that has been around for over a decade, is the most-used language for front-end web development. Over the years, JavaScript has improved and developed new capabilities to add, modify, remove, and completely change Document Object Model (DOM) elements on a website.
With these changes, JavaScript has incorporated new functionality and syntax that developers can use to improve their code. Since developers all write code a little differently, it can be challenging for other developers to jump into a project and quickly digest the current code. This transforms simple tasks such as maintenance on sites or simple modifications into a Sherlock Holmes-esque mystery.
So, how do you know if you’re coding the right way? And, who determines how to write JavaScript? This is where ECMA Script comes to the rescue.
What is ECMA Script?
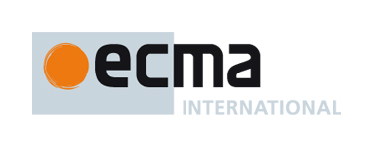
ECMA Script is a scripting language specification standardized by ECMA International in ECMA-262 and ISO/IEC 16262. It was first released in June 1997 and has had nine editions since then—releasing one version each year since 2015, when the 6th edition was released.
The main purpose of ECMA Script is to standardize scripting languages for JavaScript, as well as other languages like JScript and ActionScript. This specification is mostly used on client-side scripting for web development, however, it also works as a specification on server-side applications and services made on NodeJS.
Why should you use ECMA Script?
ECMA Script has been around for several years and has been accepted by most web developers that work with JavaScript. The way this standard specifies writing code and the improvements that it has jumping from ES5 to ES6 will help you produce cleaner, and much more powerful code. Furthermore, it can help to improve most of the code created in ES5, making legacy code easier to maintain, read, and work with.
Is ECMA Script compatible with all browsers?
The short answer is yes—for the most part. Since 2015, most of the popular browsers have adopted this standard and made changes to their compilers so they can use it without a problem.
However, the problem is that not all of the features on the latest versions of ECMA Script have been adopted by all browsers. For more information, check out these charts for ES5, ES6 and ES7+ (2016 and up). They include which ones are compatible with the majority of browsers today.
Differences between ES5 and ES6
Here are some of the improvements ES6 brought:
- Arrows
- Classes
- Enhanced object literals
- Template strings
- Destructuring
- Let + const
- Iterators + for..of
- Generators
- Unicode
- Modules
- Module loaders
- Proxies
- Symbols
- Promises
- Math + number + string + array + object APIs
- Binary and octal literals
- Reflect api
- Tail calls
To understand the true difference between ES5 and ES6, I’ve listed some examples below that show the main differences between the old and the new version of the ECMA Script standard.
String templates
ES5
var customer = { name: "Foo" };
var card = { amount: 7, product: "Bar", unitprice: 42 };
var message = "Hello " + customer.name + ",\n" +
"want to buy " + card.amount + " " + card.product + " for\n" +
"a total of " + (card.amount * card.unitprice) + " bucks?";
ES6
let customer = { name: "Foo" }
let card = { amount: 7, product: "Bar", unitPrice: 42 }
let message = `Hello ${customer.name},
want to buy ${card.amount} ${card.product} for
a total of ${card.amount * card.unitPrice} bucks?`
Binary and octal literal
ES5
parseInt("111110111", 2) === 503;
parseInt("767", 8) === 503;
0767 === 503; // only in non-strict, backward compatibility mode
ES6
0b111110111 === 503
0o767 === 503
Method properties
ES5
obj = {
foo: function (a, b) {
...
},
bar: function (x, y) {
...
},
// quux: no equivalent in ES5
...
};
ES6
obj = {
foo (a, b) {
...
},
bar (x, y) {
...
},
*quux (x, y) {
...
}
}
Class definition
ES5
var Shape = function (id, x, y) {
this.id = id;
this.move(x, y);
};
Shape.prototype.move = function (x, y) {
this.x = x;
this.y = y;
};
ES6
class Shape {
constructor (id, x, y) {
this.id = id
this.move(x, y)
}
move (x, y) {
this.x = x
this.y = y
}
}
As you can see, there are a lot of improvements from ES5 to ES6. Most of these improvements didn’t exist or were a lot harder to create, which meant more time spent on the same objective.
ECMA Script is improving with each version they release, giving developers the ability to use JavaScript in a simpler way in addition to standardizing code so anyone can understand it easily.
If you’re still not convinced that ECMA Script is a good standard, check out this full comparison of ES5 and ES6, where you’ll notice a great difference between the old syntax and the new syntax.
Which version of ECMA Script should you use?
The biggest change came in the ES6 (2015) version. Any newer version will only add “features” to the ES6 standard. However, although ES6 has great capabilities, not all of them are compatible with all browsers. In order to code using ES6+ as a standard for old browsers (like Internet Explorer), you will need a compiler like Babel, which will translate the standardized code to a compatible version of the old ES5, so all browsers can understand and compile it.
My point of view
ECMA Script does a great job defining how JavaScript should be used, and most developers seem to love it. I personally use it on every project I work on and use Babel to make it compatible with older browsers (which are sadly still used nowadays). I think this standard will stay active for a while as more developers continue to use and rely on it.
If you’re not already using ES6 on your projects, you’re missing out on new tools and elements that can provide a better coding experience. As we say in my culture “No temas a perder con un flush en mano” which translates to “Don’t worry about losing with a flush in hand.” Or, in other words, the odds of a negative outcome are low, so just go for it.